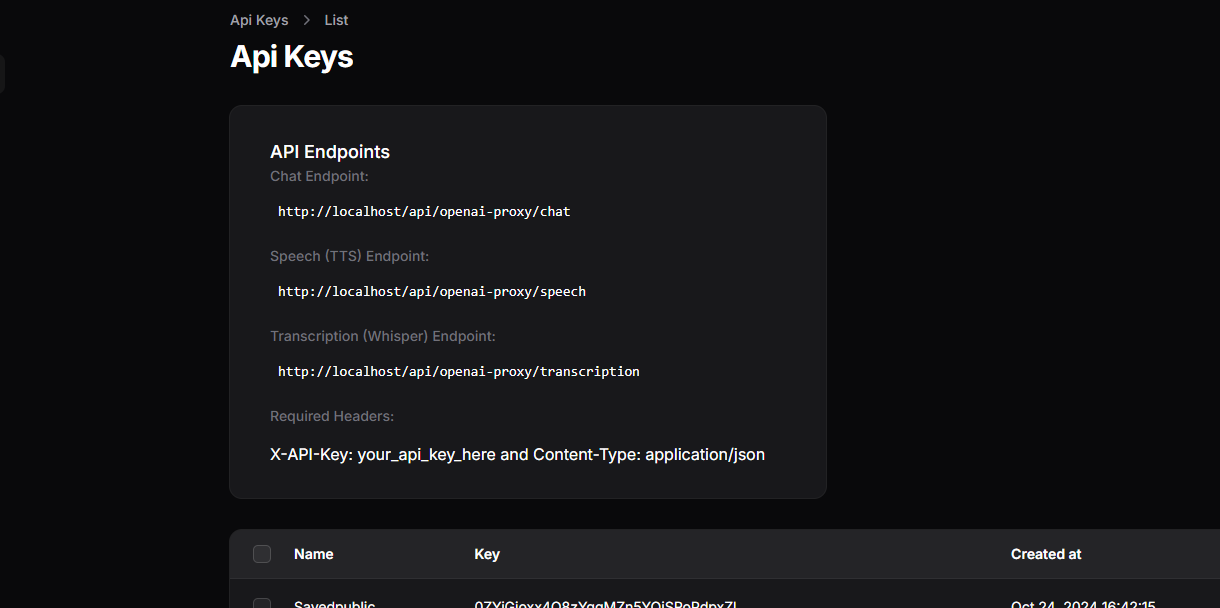
Building a Laravel OpenAI Proxy: Complete Regional Bypass Solution
Master the implementation of a secure OpenAI API proxy using Laravel 11. This comprehensive guide covers everything from basic setup to advanced features, including chat completion, text-to-speech, and transcription capabilities.
Learn how to create a secure OpenAI API proxy with Laravel 11. Step-by-step tutorial on implementing chat, speech, and transcription endpoints with regional bypass capabilities.
In today’s rapidly evolving technological landscape, having a robust and secure way to interact with powerful AI services such as OpenAI’s API is essential for developers and organizations alike. This guide is designed to take you through the process of building a secure OpenAI API proxy using Laravel 11, ensuring that you not only grasp the fundamentals but also dive into advanced features that can enhance your applications.
By the end of this guide, you will have a solid understanding of how to build a secure OpenAI API proxy using Laravel 11. You will be equipped not only with the foundational skills needed for basic API requests but also with the knowledge to implement advanced functionalities like chat completion, text-to-speech, and transcription. Whether you are an individual developer looking to harness the power of artificial intelligence in your applications or part of a larger team aiming to introduce innovative features, this guide will serve as a valuable resource in your journey.
Prepare to take your API integration skills to the next level and unlock the potential of OpenAI’s services within a secure and efficient framework.
Table of contents
Introduction to OpenAI API Regional Bypass
Creating a regional bypass solution for OpenAI API is crucial for developers in regions with limited API access. This tutorial will guide you through building a secure proxy service using Laravel 11.
Total time needed: 3 hours
Tools needed:
- PHP 8.2 or higher
- Composer
- Laravel 11
- Database (MySQL/PostgreSQL)
- Code editor
- Terminal/Command prompt
Materials needed:
- OpenAI API key
- Composer packages
- Laravel project files
- Database credentials
Project Setup and Configuration
Step 1: Initial Setup
# Create new Laravel project
composer create-project laravel/laravel openai-proxy
# Install required packages
composer require openai-php/client
composer require guzzlehttp/guzzle
composer require filament/filament:"^3.0-stable"
Step 2: Environment Configuration
OPENAI_API_KEY=your_api_key_here
APP_URL=your-domain.com
Core Components Implementation
1. API Key Management
// app/Models/ApiKey.php
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
use Illuminate\Support\Str;
class ApiKey extends Model
{
protected $fillable = ['name', 'key'];
public static function generateUniqueKey(): string
{
return 'pk_' . Str::random(32);
}
}
2. OpenAI Proxy Controller
// app/Http/Controllers/OpenAIProxyController.php
namespace App\Http\Controllers;
use OpenAI;
use Illuminate\Http\Request;
class OpenAIProxyController extends Controller
{
private function initializeOpenAI($apiKey)
{
return OpenAI::client($apiKey);
}
public function proxyChat(Request $request)
{
$client = $this->initializeOpenAI(config('services.openai.key'));
return $client->chat()->create([
'model' => $request->input('model', 'gpt-3.5-turbo'),
'messages' => $request->input('messages'),
]);
}
}
Feature Implementation Guide
1. Chat Completion Implementation
public function handleChatCompletion($messages, $model = 'gpt-3.5-turbo')
{
try {
$response = $this->openai->chat()->create([
'model' => $model,
'messages' => $messages,
'temperature' => 0.7,
]);
return $response->toArray();
} catch (\Exception $e) {
return $this->handleError($e);
}
}
2. Text-to-Speech Implementation
public function handleTextToSpeech($text, $voice = 'alloy')
{
try {
$response = $this->openai->audio()->speech([
'model' => 'tts-1-hd',
'input' => $text,
'voice' => $voice,
]);
return $response;
} catch (\Exception $e) {
return $this->handleError($e);
}
}
Security Implementation
API Key Validation
private function validateApiKey($request)
{
$apiKey = $request->header('X-API-Key');
if (!$apiKey || !ApiKey::where('key', $apiKey)->exists()) {
throw new \Exception('Unauthorized', 401);
}
return true;
}
Usage Examples
JavaScript Example
const makeRequest = async (endpoint, data) => {
const response = await fetch(`${baseUrl}/api/openai-proxy/${endpoint}`, {
method: 'POST',
headers: {
'X-API-Key': 'your_api_key_here',
'Content-Type': 'application/json',
},
body: JSON.stringify(data)
});
return await response.json();
};
PHP Example
$client = new \GuzzleHttp\Client();
$response = $client->post('https://your-domain.com/api/openai-proxy/chat', [
'headers' => [
'X-API-Key' => 'your_api_key_here',
'Content-Type' => 'application/json',
],
'json' => [
'model' => 'gpt-3.5-turbo',
'messages' => [
['role' => 'user', 'content' => 'Hello!']
]
]
]);
Frequently Asked Questions
Yes, this proxy solution is legal as it simply facilitates API access while maintaining OpenAI’s terms of service.
Rate limits follow OpenAI’s standard limitations. Monitor your usage through the admin dashboard.
Yes, but ensure proper security measures and monitoring are in place.
The system includes comprehensive error handling and logging. Check the logs for troubleshooting.
Best Practices and Tips
- Error Handling
- Implement comprehensive logging
- Use try-catch blocks
- Return meaningful error messages
- Performance Optimization
- Cache responses when possible
- Implement rate limiting
- Monitor server resources
- Security Measures
- Validate all inputs
- Implement request signing
- Use HTTPS
- Regular security audits
Troubleshooting Common Issues
- API Key Issues
- Verify key format
- Check permissions
- Confirm key validity
- Connection Problems
- Check network connectivity
- Verify SSL certificates
- Monitor timeout settings
- Rate Limiting
- Implement exponential backoff
- Monitor usage patterns
- Set up alerts
This comprehensive guide should help you implement a secure and efficient OpenAI API proxy using Laravel. Remember to regularly update dependencies and monitor security advisories.
- 0Email
- 0Facebook
- 0Twitter
- 0Pinterest
- 0LinkedIn
- 0Like
- 0Digg
- 0Del
- 0Tumblr
- 0VKontakte
- 0Reddit
- 0Buffer
- 0Love This
- 0Weibo
- 0Pocket
- 0Xing
- 0Odnoklassniki
- 0WhatsApp
- 0Meneame
- 0Blogger
- 0Amazon
- 0Yahoo Mail
- 0Gmail
- 0AOL
- 0Newsvine
- 0HackerNews
- 0Evernote
- 0MySpace
- 0Mail.ru
- 0Viadeo
- 0Line
- 0Flipboard
- 0Comments
- 0Yummly
- 0SMS
- 0Viber
- 0Telegram
- 0Subscribe
- 0Skype
- 0Facebook Messenger
- 0Kakao
- 0LiveJournal
- 0Yammer
- 0Edgar
- 0Fintel
- 0Mix
- 0Instapaper
- 0Print
- Share
- 0Copy Link